接入说明
我们允许第三方接入我们的平台,调用开放 API 获取用户信息、应用信息、创建应用等。
我们的开发者平台仍然在开发中,目前您需要通过帮助菜单的技术支持提出接入申请,要求提供下列信息:
- 帐户邮箱
- 申请者的详细信息:名称(个人或者公司)、地址、联系方式(手机或者电话)等。
- 申请的第三方平台的详细信息: 名称、介绍描述、网站地址
- 期望的接入方式:详情见下文。
在申请成功后,我们会发送 accessKey 和 accessSecret 提供给您接入。
参数说明
当虹云的API,一般都会包含5个参数action,accessKey,version,timestamp 和 signature
action:api名称
accessKey:分配给用户的访问key。
version:api版本
timestamp:时间戳(1970年1月1日以来的毫秒数,格林尼治时间)
signature:请求签名,签名生成规则:对所有参数按照名称排序(CASE_INSENSITIVE_ORDER)生成一个字符串,并在字符串头上加上accessSecret,然后对该字符串调用[HmacSHA256](http://en.wikipedia.org/wiki/Hash-based_message_authentication_code) 算法生成signature。每一个API,根据输入参数的不同,生成的签名都是不同的。
我们以API:创建频道 为例来说明签名的生成,该接口的参数为accessKey,action,signature,version,timestamp,name:
accessKey = "a020e193-0f1"
accessSecret = "5GcXHNYdAVVdFW0yervG"
action = "ugcCreateChannel"
version = "2.0"
timestamp = "1466488681033"
name = "直播测试频道"
那么调用该接口的signature参数就是:
hmacSHA256Encrypt("5GcXHNYdAVVdFW0yervGaccessKey=a020e193-0f1action=ugcCreateChannelname=直播测试频道timestamp=1466488681033version=2.0", 5GcXHNYdAVVdFW0yervG)
计算后的值为
69a22291e517179e76e55ad7c21269c17462e102adf0eb0526cc5012d9b4cd9a
最终的请求为:
http://api.danghongyun.com/rest?timestamp=1466488681033&accessKey=a020e193-0f1&name=直播测试频道&action=ugcCreateChannel&signature=69a22291e517179e76e55ad7c21269c17462e102adf0eb0526cc5012d9b4cd9a&version=2.0
示例代码
HmacSha256 加密算法
SHA256 Hmac 签名生成64位字符串, 可参考如下代码:
Java:
/**
* 使用 HMAC-SHA256 签名方法对对plainText进行签名
* @param plainText 被签名的字符串
* @param accessSecret 密钥
* @return
* @throws Exception
*/
public static String hmacSHA256Encrypt(String plainText, String accessSecret) throws Exception {
Mac mac = Mac.getInstance("HmacSHA256");
byte[] secretByte = accessSecret.getBytes("UTF-8");
byte[] dataBytes = plainText.getBytes("UTF-8");
SecretKey secret = new SecretKeySpec(secretByte, "HMACSHA256");
mac.init(secret);
byte[] doFinal = mac.doFinal(dataBytes);
byte[] hexB = new Hex().encode(doFinal);
String signature = new String(hexB);
return signature;
}
PHP:
<?php
echo hash_hmac('sha256', $plainText, $accessSecret);
?>
C#:
private static string hmacSHA256Encrypt(string plainText, string accessSecret)
{
HMACSHA256 hash = new HMACSHA256(Encoding.UTF8.GetBytes(accessSecret));
byte[] bytes = hash.ComputeHash(Encoding.UTF8.GetBytes(plainText));
return BitConverter.ToString(bytes).Replace("-", string.Empty).ToLower();
}
签名生成
Java:
public static String generateSignature(HashMap<String, Object> param, String secret) throws Exception {
if (StringUtils.isBlank(secret) || MapUtils.isEmpty(param)) {
return null;
}
List<String> keyList = new ArrayList<String>(param.keySet());
Collections.sort(keyList, String.CASE_INSENSITIVE_ORDER);
StringBuilder sb = new StringBuilder(secret);
for (String key : keyList) {
if (param.get(key) != null) {
if (!key.equals("signature")) {
sb.append(key);
sb.append("=");
sb.append(param.get(key));
}
}
}
String signature = hmacSHA256Encrypt(sb.toString(), secret);
return signature;
}
PHP:
function mkSig(array $arr, $accessKey, $accessSecret){
ksort($arr);
$request=$accessSecret;
foreach ($arr as $key => $value) {
$request .= $key.'='.$value;
}
echo "\n",$request,"\n";
return sha256($request, $accessSecret);
}
C#:
public static string generateSignature(IDictionary<string,object> param, string secret)
{
List<string> keys = new List<string>(param.Keys);
keys.Sort(StringComparer.OrdinalIgnoreCase);
StringBuilder sb = new StringBuilder(secret);
foreach (string key in keys)
{
sb.Append(key).Append("=").Append(param[key]);
}
return hmacSHA256Encrypt(sb.ToString(), secret);
}
API 调用
Java:
String ACCESSKEY = "a020e193-0f1";
String ACCESSSECRT = "5GcXHNYdAVVdFW0yervG";
int userId = 1;
String URI = "http://api.danghongyun.com/rest?" ;
HashMap<String,String> params = new HashMap<String,String>();
params.put("accessKey", ACCESSKEY);
params.put("signature", generateSignature(params, ACCESSSECRT));
String request = createURL(URI, params);
String httpReslut = HttpUtil.get(request);
System.out.println(httpReslut);
return JSON.parseArray(httpReslut);
PHP:
Url拼装:
function mkUrl(array $dataArr){
$url='';
foreach ($dataArr as $key => $value) {
$url .= $key.'='.$value.'&';
}
return substr($url, 0, strlen($url)-1);
}
Get:
function curlGet($url){
echo "\n",$url,"\n";
$ch = curl_init($url) ;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_BINARYTRANSFER, true);
$res = curl_exec($ch);
curl_close($ch);
return $res;
}
Post:
function curlPost(array $dataArr, $url){
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL,$url);
curl_setopt($ch, CURLOPT_POST,count($dataArr));
curl_setopt($ch, CURLOPT_POSTFIELDS,$dataArr);
ob_start();
curl_exec($ch);
$res = ob_get_contents();
ob_end_clean();
curl_close($ch);
return $res;
}
C#:
// add parameters
SortedList<string, string> sl = new SortedList<string, string>();
sl.Add("accessKey", txtAccessKey.Text);
// caculate signature
string signature = generateSignature(sl, txtAccessSecret.Text);
sl.Add("signature", signature );
// make url
string url = createUrl(txtUri.Text, sl);
// invoke and get result
WebRequest request = WebRequest.Create(url);
try
{
WebResponse wResp = request.GetResponse();
Stream respStream = wResp.GetResponseStream();
using (StreamReader reader = new StreamReader(respStream, Encoding.UTF8))
{
Console.WriteLine(reader.ReadToEnd());
}
} catch(Exception e1) {
Console.WriteLine(e1.Message);
}
约定
- 所有接口使用http协议进行交互
- 请求方法为POST的接口请将Http header中的Content-Type设置为x-www-form-urlencoded
- 所有接口的返回数据格式为json, code为0时表示操作成功,其它值表示操作失败,失败原因可以通过message获取
直播调用时序图
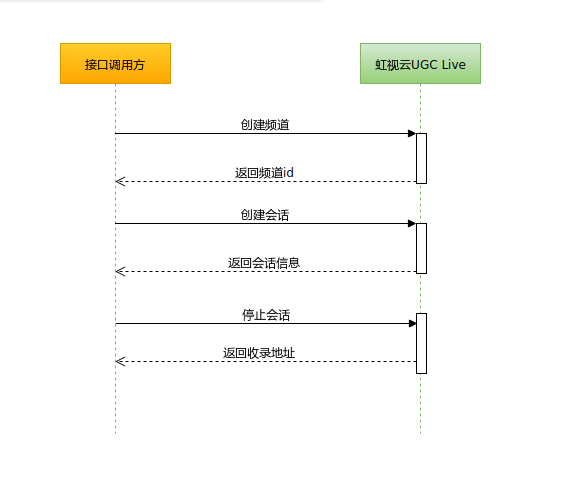
频道相关
创建频道
服务地址:
http://api.danghongyun.com/rest
请求方式:
POST
接收参数:
参数 | 描述 | 数据类型 | 必选/可选 |
---|---|---|---|
accessKey | 用户访问key | String | 必选 |
action | api名称,此接口为ugcCreateChannel | String | 必选 |
version | api版本,目前必须为 2.0 | String | 必选 |
signature | 签名 | String | 必选 |
timestamp | api调用时间戳,1970年1月1日以来的毫秒数,如1466488681033 | String | 必选 |
name | 频道名称 | String | 必选 |
返回数据:
{
code: 0,
message: "",
result: {
id: 1
}
}
返回数据描述:
名称 | 描述 |
---|---|
id | 频道id |
修改频道
服务地址:
http://api.danghongyun.com/rest
请求方式:
POST
接收参数:
参数 | 描述 | 数据类型 | 必选/可选 |
---|---|---|---|
accessKey | 用户访问key | String | 必选 |
action | api名称,此接口为ugcUpdateChannel | String | 必选 |
version | api版本,目前必须为 2.0 | String | 必选 |
signature | 签名 | String | 必选 |
timestamp | api调用时间戳,1970年1月1日以来的毫秒数,如1466488681033 | String | 必选 |
id | 频道id | long | 必选 |
name | 频道名称 | String | 必选 |
返回数据:
{
code: 0,
message: ""
}
获取频道信息
服务地址:
http://api.danghongyun.com/rest
请求方式:
GET
接收参数:
参数 | 描述 | 数据类型 | 必选/可选 |
---|---|---|---|
accessKey | 用户访问key | String | 必选 |
action | api名称,此接口为ugcGetChannel | String | 必选 |
version | api版本,目前必须为 2.0 | String | 必选 |
signature | 签名 | String | 必选 |
timestamp | api调用时间戳,1970年1月1日以来的毫秒数,如1466488681033 | String | 必选 |
id | 频道id | long | 必选 |
返回数据:
{
code: 0,
message: "",
result: {
status: 0,
current_session: {
"id": 14,
"status": 0,
"play": "rtmp://play-server/xxx",
"push": "rtmp://push-server/xxx",
"url": null,
"hls": "http://hls-server/index.m3u8",
"thumbnail": "http://xxxxx.com/thumbnail.jpg"
},
id: 1
}
}
返回数据描述:
result
名称 | 描述 |
---|---|
id | 频道id |
status | 请参考频道状态 |
current_session | 当前激活的会话信息,如果没有 |
current_session
名称 | 描述 |
---|---|
id | 会话id |
status | 请参考会话状态 |
url | 收录文件播放地址,当状态为停止时才返回该地址 |
push | 推流地址,当状态非停止时才会有值 |
play | rtmp播放地址,当状态非停止时才会有值 |
hls | hls播放地址,一般用于在html5中播放,当状态非停止时才会有值 |
thumbnail | 最近一张截图的url |
删除频道
服务地址:
http://api.danghongyun.com/rest
请求方式:
POST
接收参数:
参数 | 描述 | 数据类型 | 必选/可选 |
---|---|---|---|
accessKey | 用户访问key | String | 必选 |
action | api名称,此接口为ugcDeleteChannel | String | 必选 |
version | api版本,目前必须为 2.0 | String | 必选 |
signature | 签名 | String | 必选 |
timestamp | api调用时间戳,1970年1月1日以来的毫秒数,如1466488681033 | String | 必选 |
id | 频道id | long | 必选 |
返回数据:
{
code: 0,
message: ""
}
屏蔽频道
屏蔽频道时将自动停止关联到频道的会话;另外,当频道处于屏蔽状态时,将无法创建会话
服务地址:
http://api.danghongyun.com/rest
请求方式:
POST
接收参数:
参数 | 描述 | 数据类型 | 必选/可选 |
---|---|---|---|
accessKey | 用户访问key | String | 必选 |
action | api名称,此接口为ugcBlockChannel | String | 必选 |
version | api版本,目前必须为 2.0 | String | 必选 |
signature | 签名 | String | 必选 |
timestamp | api调用时间戳,1970年1月1日以来的毫秒数,如1466488681033 | String | 必选 |
id | 频道id | long | 必选 |
返回数据:
{
code: 0,
message: ""
}
恢复频道
恢复已屏蔽的频道
服务地址:
http://api.danghongyun.com/rest
请求方式:
POST
接收参数:
参数 | 描述 | 数据类型 | 必选/可选 |
---|---|---|---|
accessKey | 用户访问key | String | 必选 |
action | api名称,此接口为ugcRestoreChannel | String | 必选 |
version | api版本,目前必须为 2.0 | String | 必选 |
signature | 签名 | String | 必选 |
timestamp | api调用时间戳,1970年1月1日以来的毫秒数,如1466488681033 | String | 必选 |
id | 频道id | long | 必选 |
返回数据:
{
code: 0,
message: ""
}
会话相关
创建会话
创建一个会话,用于推流,播放及收录.
注意: 如果当前频道已存在激活的会话,将直接返回当前激活的会话信息,否则将创建一个新的会话
服务地址:
http://api.danghongyun.com/rest
请求方式:
POST
接收参数:
参数 | 描述 | 数据类型 | 必选/可选 |
---|---|---|---|
accessKey | 用户访问key | String | 必选 |
action | api名称,此接口为ugcCreateChannelSession | String | 必选 |
version | api版本,目前必须为 2.0 | String | 必选 |
signature | 签名 | String | 必选 |
timestamp | api调用时间戳,1970年1月1日以来的毫秒数,如1466488681033 | String | 必选 |
id | 频道id | long | 必选 |
返回数据:
{
code: 0,
message: "",
result: {
id: 1,
status: 0,
push: "rtmp://xxxx",
play: "rtmp://xxxxx",
hls: "http://hls-server/index.m3u8"
}
}
返回描述:
result
名称 | 描述 |
---|---|
id | 会话id |
status | 请参考会话状态 |
push | 推流地址 |
play | 播放地址 |
hls | hls播放地址 |
获取会话信息
服务地址:
http://api.danghongyun.com/rest
请求方式:
GET
接收参数:
参数 | 描述 | 数据类型 | 必选/可选 |
---|---|---|---|
accessKey | 用户访问key | String | 必选 |
action | api名称,此接口为ugcGetChannelSession | String | 必选 |
version | api版本,目前必须为 2.0 | String | 必选 |
signature | 签名 | String | 必选 |
timestamp | api调用时间戳,1970年1月1日以来的毫秒数,如1466488681033 | String | 必选 |
id | 会话id | long | 必选 |
返回数据:
{
code: 0,
message: "",
result: {
"id": 14,
"status": 0,
"play": "rtmp://play-server/xxx",
"push": "rtmp://push-server/xxx",
"url": null,
"hls": "http://hls-server/index.m3u8"
}
}
返回描述
result
名称 | 描述 |
---|---|
id | 会话id |
status | 请参考会话状态 |
push | 推流地址,当状态非停止时才会有值 |
play | rtmp播放地址,当状态非停止时才会有值 |
url | 收录播放地址,当状态为停止时才会有值 |
hls | hls播放地址,一般用于在html5中播放,当状态非停止时才会有值 |
停止会话
停止会话后将返回收录文件播放地址
服务地址:
http://api.danghongyun.com/rest
请求方式:
POST
接收参数:
参数 | 描述 | 数据类型 | 必选/可选 |
---|---|---|---|
accessKey | 用户访问key | String | 必选 |
action | api名称,此接口为ugcStopChannelSession | String | 必选 |
version | api版本,目前必须为 2.0 | String | 必选 |
signature | 签名 | String | 必选 |
timestamp | api调用时间戳,1970年1月1日以来的毫秒数,如1466488681033 | String | 必选 |
id | 会话id | long | 必选 |
返回数据:
{
code: 0,
message: "",
result: {
url: "http://xxxxx"
}
}
返回描述:
result
名称 | 描述 |
---|---|
url | 收录播放地址 |
删除会话
服务地址:
http://api.danghongyun.com/rest
请求方式:
POST
接收参数:
参数 | 描述 | 数据类型 | 必选/可选 |
---|---|---|---|
accessKey | 用户访问key | String | 必选 |
action | api名称,此接口为ugcDeleteChannelSession | String | 必选 |
version | api版本,目前必须为 2.0 | String | 必选 |
signature | 签名 | String | 必选 |
timestamp | api调用时间戳,1970年1月1日以来的毫秒数,如1466488681033 | String | 必选 |
id | 会话id | long | 必选 |
返回数据:
{
code: 0,
message: ""
}
附录
频道状态
值 | 描述 |
---|---|
0 | 启用 |
1 | 禁用 |
会话状态
值 | 描述 |
---|---|
0 | 未就绪 |
1 | 直播中 |
2 | 停止 |
3 | 断流.当超过断流最大时间限制后,会话将自动停止 |